create-react-appは、Facebook公式のツールで、Reactプロジェクトを構築してくれます。ビルドツール( Webpack
Babel
など)の面倒な設定をスキップして、すぐにコーディング作業を進めることができます。ここでは、create-react-appを利用したReactプロジェクトの構築方法を確認します。
目次
作業環境
OSはMacです。以下の環境で作業します。
$ node -v
v12.15.0
$ npm -v
6.13.4
$ yarn -v
1.22.4
create-react-app helpを確認
create-react-app --help
で主な利用方法を確認できます。
$ npx create-react-app --help
npx: installed 63 in 3.896s
Usage: create-react-app <project-directory> [options]
Options:
-V, --version output the version number
--verbose print additional logs
--info print environment debug info
--scripts-version <alternative-package> use a non-standard version of react-scripts
--use-npm
--use-pnp
--typescript
-h, --help output usage information
Only <project-directory> is required.
A custom --scripts-version can be one of:
- a specific npm version: 0.8.2
- a specific npm tag: @next
- a custom fork published on npm: my-react-scripts
- a local path relative to the current working directory: file:../my-react-scripts
- a .tgz archive: https://mysite.com/my-react-scripts-0.8.2.tgz
- a .tar.gz archive: https://mysite.com/my-react-scripts-0.8.2.tar.gz
It is not needed unless you specifically want to use a fork.
If you have any problems, do not hesitate to file an issue:
https://github.com/facebook/create-react-app/issues/new
新規Reactプロジェクトを構築
create-react-appの使い方
create-react-app
を利用して、新規プロジェクト( my-app
)を構築します。npx
コマンドを利用すれば、npmパッケージの「ダウンロード」と「実行」をまとめて行なってくれます。
npx create-react-app my-app
TypeScriptアプリの作成
TypeScriptで記述したい場合、--template typescript
オプションを指定します。
$ npx create-react-app my-app --template typescript
npx: installed 92 in 3.547s
Creating a new React app in /xxxx/my-app.
Installing packages. This might take a couple of minutes.
Installing react, react-dom, and react-scripts with cra-template-typescript...
(省略)
Success! Created my-app at /xxxx/my-app
Inside that directory, you can run several commands:
yarn start
Starts the development server.
yarn build
Bundles the app into static files for production.
yarn test
Starts the test runner.
yarn eject
Removes this tool and copies build dependencies, configuration files
and scripts into the app directory. If you do this, you can’t go back!
We suggest that you begin by typing:
cd my-app
yarn start
Happy hacking!
react
react-dom
react-scripts
とTypeScriptに必要なパッケージがインストールされました。
- react
- React本体です。
- react-dom
- react-scripts
- ビルドツールなどの面倒を見てくれます。
package.json
のscripts
にreact-scriptsのコマンドが設定されます。- https://github.com/facebook/create-react-app/tree/master/packages/react-scripts
開発環境立ち上げ
作成した環境に移動して、開発環境を立ち上げてみます。
$ cd my-app
$
$ yarn start
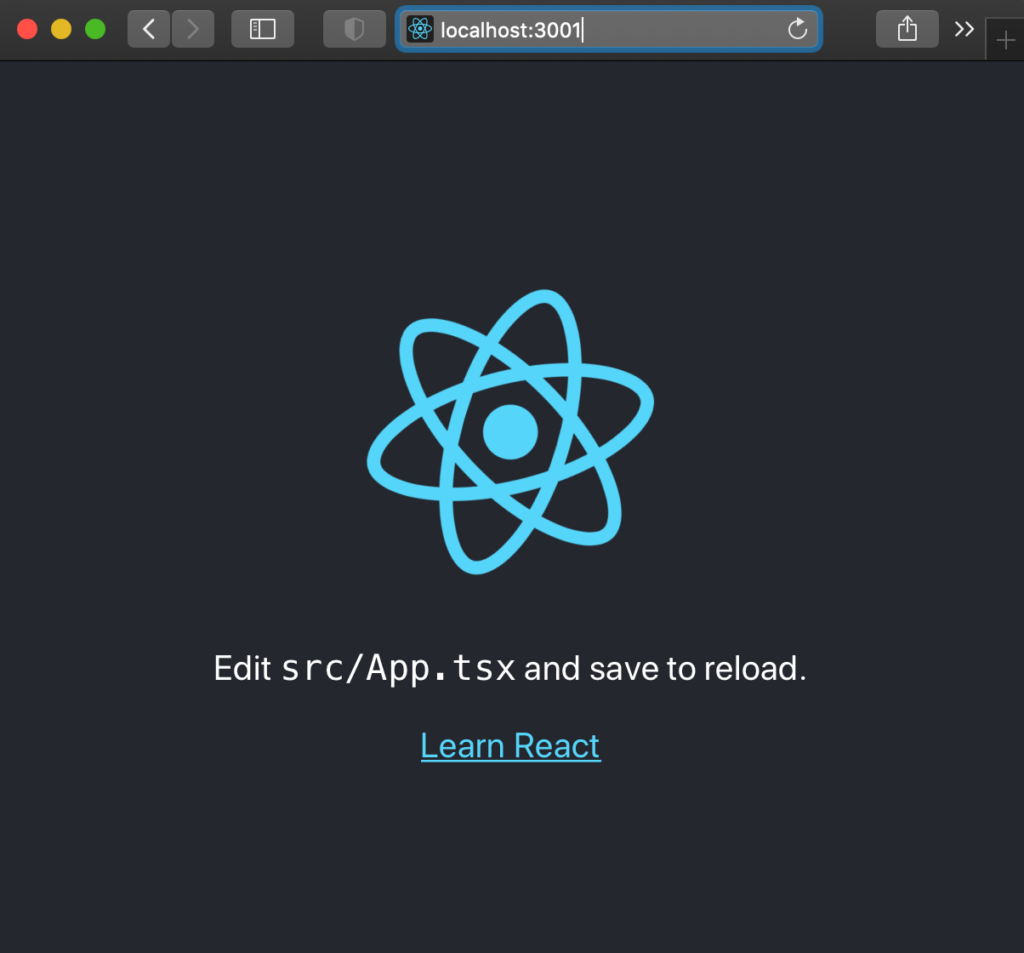
すでに3000ポートを利用していたので、代わりに3001ポートが利用されました。
フォルダ構成を確認
(base) $ tree -I node_modules
.
├── README.md
├── package.json
├── public
│ ├── favicon.ico
│ ├── index.html
│ ├── logo192.png
│ ├── logo512.png
│ ├── manifest.json
│ └── robots.txt
├── src
│ ├── App.css
│ ├── App.test.tsx
│ ├── App.tsx
│ ├── index.css
│ ├── index.tsx
│ ├── logo.svg
│ ├── react-app-env.d.ts
│ ├── reportWebVitals.ts
│ └── setupTests.ts
├── tsconfig.json
└── yarn.lock
2 directories, 19 files
package.jsonを確認
package.json
の記述を確認します。
{
"name": "my-app",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.11.4",
"@testing-library/react": "^11.1.0",
"@testing-library/user-event": "^12.1.10",
"@types/jest": "^26.0.15",
"@types/node": "^12.0.0",
"@types/react": "^16.9.53",
"@types/react-dom": "^16.9.8",
"react": "^17.0.1",
"react-dom": "^17.0.1",
"react-scripts": "4.0.0",
"typescript": "^4.0.3",
"web-vitals": "^0.2.4"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
}
}
src/index.tsxを確認
src/index.tsx
の記述を確認します。
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
public/index.html
のid属性がrootの要素内にReactを描画するよう記述されています。
React.StrictMode
で <App />
を囲むことにより、アプリケーションの潜在的な問題をチェックしてくれます。